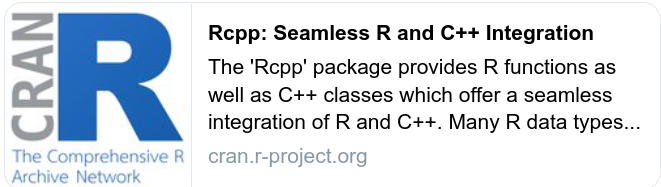
Rcpp: Seamless R and C++ Integration
Extending and growing R applications via an easy-to-use, robust, and performant C++ interface.
All key R data types map naturally into key C++ data structures, and simple interfaces make transfer back and forth very easy. And by composing more complex interfaces become possible.
Numerous examples, as well as extensive documentation, facilitate its use.